Lab 2 : Single Cycle CPU w/ Branch Instruction#
Introduction#
In previous lab requirement, only some basic instruction are implemented. For lab2, we are going to make our CPU more powerful, which support more RV32I standard instructions. To reach this goal, you might notice architecture modification is necessary.
The architecture of lab1 doesn’t support jump reletaed insturctions.
After finished lab2, you will figure out you own solution to make
original CPU support jal
and jalr
instructions.
bne
,blt
,bge
are easier, you can first try realize them.
Lab Source Code#
TAs think you should finish your lab2 based on your lab1 submission, so there is no template after lab1.
Single Cycle CPU#
Architecture#
You can follow the architecture graph to realize a single cycle CPU, however, this architecture doesn’t consider jump related instructions.
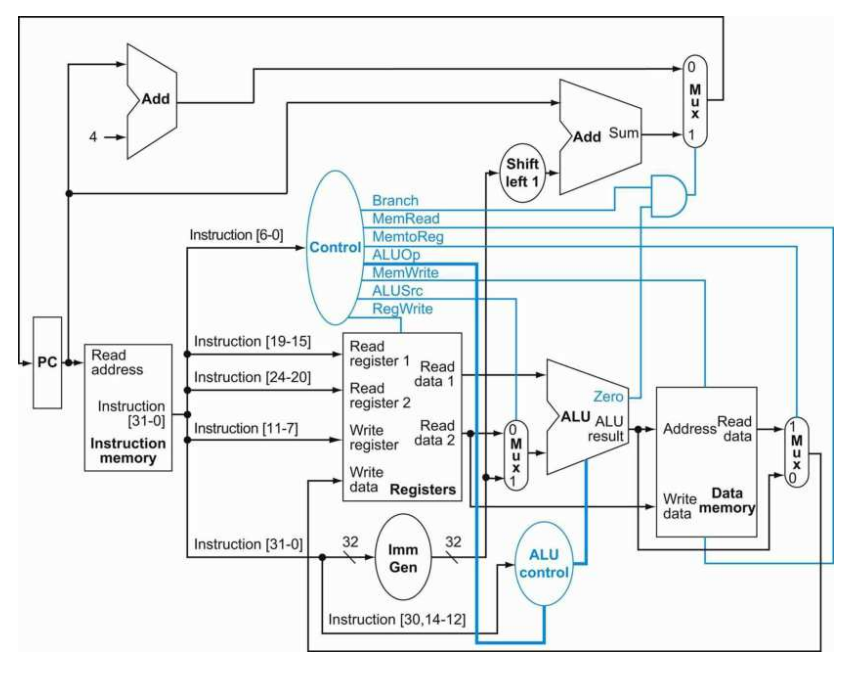
Operation#
Hint
All operation are signed
jal
jal
storespc+4
inregs[rd]
, executespc = pc + imm << 1
jalr
jalr
storespc+4
inregs[rd]
, execturespc = regs[rs1] + imm
bne
blt
bge
Requirement#
Implement your RISC-V single cycle CPU, your CPU should be able to support following RISC-V ISA
add, addi, sub, and, andi, or, ori
slt, slti
lw, sw, beq
jal, jalr, bne, blt, bge
TAs have prepare verilator testbench and some TEST_INSTRUCTION.txt
to grade your design. We will verify correctness by comparing register
value, so
Warning
Don’t modify register, instruction memory and CPU interface, or you will get 0 points.
Warning
Reset signal rst
should be active low.
Warning
Your design must satisfy lab1 requirement.
Warning
You must follow this ISA table to implement.
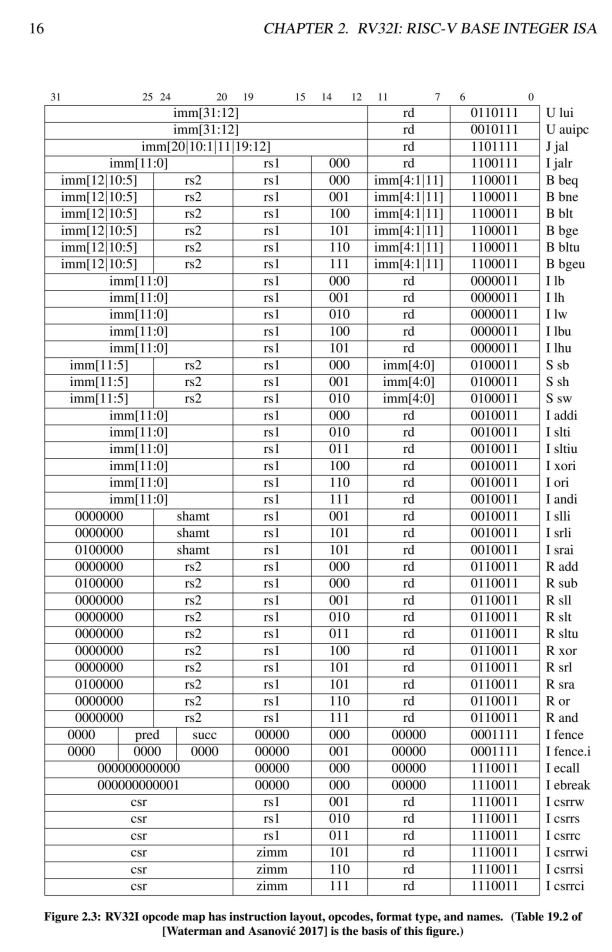
Submission#
Please submit your source code as zip file to E3.
The name of the zip file should be <student_id>.zip, and the structure of the file should be as the following:
<stduent_id>.zip
|- <student_id>/
|- ...(your source codes)
Warning
The deadline is on 4/11 23:59.
Hint#
Read textbook first, understand each submodule’s functionality.
Debugging with waveform makes your life easier.
Try to generate your own risc-v machine code with Ripe, you can write simple assembly to verify if your code runs as expect.
Reference#
Computer Organization and Design RISC-V Edition, CH4